What are Pointers in C Programming: Concepts, Best Practices, and Examples
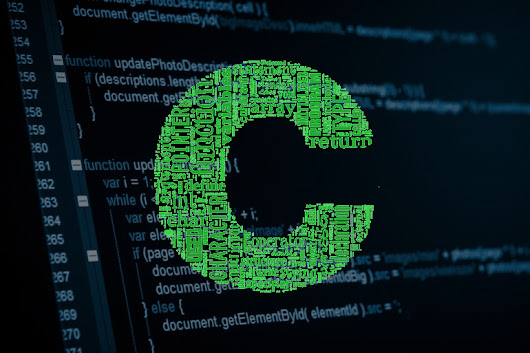
1. Introduction Pointers are one of the most powerful features of the C programming language. Pointers are variables that hold the memory address of another variable. In this tutorial, we'll explore the basics of pointers, why they are used, and how they work. 1.1. What are Pointers? A pointer is a variable that stores the memory address of another variable. Pointers allow you to manipulate data indirectly, by accessing the memory address of the data, rather than the data itself. 1.2. Why use Pointers? Pointers are used for a variety of reasons, including: Dynamic memory allocation: Pointers allow you to dynamically allocate memory at runtime, which is useful for creating data structures such as linked lists and trees.